※ 스파르타 코딩클럽의 웹개발 종합반(5주)을 공부하며 기억하고 싶은 특이점들만 기록, 정리해 놓은 노트이다.
Flask 기초 1탄 - 구구절절한 연습
Flask 기초 1탄 - 구구절절한 연습
Flask 프레임워크 : 서버를 구동시켜주는 편한 파이썬 코드 모음.
Flask 시작하기
- 파이참에서 새 파이썬 프로젝트를 생성한다. (가상환경 설정까지 포함)
- 만들어진 프로젝트 폴더에서 Flask 패키지를 설치해준다. (‘파일 → 옵션’에 들어가 Python Interpreter → + 클릭 → ‘flask’ 검색 후 설치)
- 만들어진 프로젝트 폴더 안에 templates 폴더와 static 폴더, app.py 파일을 만들어둔다. (=Flask 프로젝트 기본 구조)
- app.py 파일에 시작 템플릿을 복붙한다.
Flask 시작 템플릿
# app.py파일 from flask import Flask app = Flask(__name__) @app.route('/') def home(): return 'This is Home!' @app.route('/mypage') def mypage(): return 'This is Mypage~~!!!' # => app.route 내의 주소도 다르게, 함수명도 다르게 해야 다른 url 페이지를 만드는 것이 됨 주의. if __name__ == '__main__': app.run('0.0.0.0',port=5000, debug=True)
- Run을 눌러서 파일을 실행하고 ‘서버가 실행중~’이라는 문구를 콘솔 창에서 확인한다.
- 이제 브라우저 창을 켜고 웹주소
localhost:5000
과localhost:5000/mypage
로 접근할 수 있다. (= 로컬 개발환경)
- 서버를 종료하려면 다시 콘솔창에서 Ctrl + C로 파일 실행을 종료해준다.
Flask 프로젝트 기본 폴더구조
Flask 서버를 만들 때 프로젝트 폴더 안에 항상 ㄴstatic 폴더 (이미지, css파일을 넣어둡니다) ㄴtemplates 폴더 (html파일을 넣어둡니다) ㄴapp.py 파일
이렇게 세 가지를 만들어 둔다.
웹주소에 HTML 페이지 연결하기
웹주소에 HTML 페이지 연결하기
위의 기본 Flask 템플릿 중에서 HTML코드를 넣을 수 있는 부분이 있다:
@app.route('/')
def home():
return 'This is Home!' # => 이부분을 HTML 태그로 바꾸면 HTML 코드 그대로 (잘 해석되어) 적용된다!
예를 들면
@app.route('/')
def home():
return '<button>버튼입니다. HTML 처리되어 진짜 버튼이 나오지요</button>'
이렇게 해주면 localhost:5000
페이지에서 이렇게 뜨게 된다:

그렇다면 저 return
자리에 내가 보이고 싶은 HTML 코드를 넣으면 내가 보여주고 싶은 페이지를 보여줄 수 있겠다는 얘기가 된다. 두 가지 처리만 하면 된다:
templates
폴더에index.html
과 같은 웹 페이지 파일을 넣어두기
app.py
(내 Flask 기본 템플릿)에 flask 내장함수render_template
적용하기# app.py파일 from flask import Flask, render_template app = Flask(__name__) ## URL 별로 함수명이 같거나, ## route('/') 등의 주소가 같으면 안됩니다. @app.route('/') def home(): return render_template('index.html') if __name__ == '__main__': app.run('0.0.0.0', port=5000, debug=True)
Flask - GET 요청과 응답
Flask - GET 요청과 응답
기본적으로 다음의 두 단계를 처리해주면 된다:
- 프론트 쪽에 GET 요청을 보내는 코드를 짜고
- 서버 쪽에 GET 요청을 확인하고 처리하는 코드를 짜주기
1. 프론트(index.html)에 Ajax로 GET 요청 심어주기
1. 프론트(index.html)에 Ajax로 GET 요청 심어주기
- index.html 파일에 jQuery 임포트
- 임의의 버튼에
onclick='hey()'
를 설정해주기
hey()
함수 정의하기 -$.ajax()
로 GET / POST 요청하기<script> function hey() { $.ajax({ type: "GET", url: "/test?title_give=봄날은간다", data: {}, success: function (response) { console.log(response) } }) } </script> ... <body> <button onclick="hey()">나는 버튼!</button> </body>
해석:
‘/test’라는 외부 창구에, 약속된 ‘title_give’라는 항목에 대응되는 값으로 ‘봄날은간다’를 가지고 조회를 요청(GET)함.
조회가 성공적으로 이루어지면 실행하는 함수는 콘솔창에 그 응답받은 것을 출력한다.
‘그 응답받은 것’이란,
@app.route('/test', methods=['GET'])
로 꾸밈받은 서버쪽 함수가 return해주는 값! 즉, 여기서response
={'result':'success', 'msg': '이 요청은 GET!'}
인 것이다.HTML쪽의 콘솔창이란 웹 브라우저의 개발자 도구 콘솔창을 말함.
버튼을 클릭하면 이 모든 일이 일어난다.
2. 서버(app.py)에 GET 요청 대응 API코드 만들기
2. 서버(app.py)에 GET 요청 대응 API코드 만들기
- app.py 파일에
from flask import request, jsonify
까지 추가로 임포트하기
- GET 요청 확인 템플릿 추가하기:
@app.route('/test', methods=['GET']) def test_get(): title_receive = request.args.get('title_give') print(title_receive) return jsonify({'result':'success', 'msg': '이 요청은 GET!'})
해석:
이 함수(처리)는 ‘/test’라는 창구에서 이루어지고 있다.
‘title_give’라는 약속된 양식(key)에 딸려온 값을 받아서 title_receive라는 변수에 저장했다.
저장한 값을 출력했다. 이 때는 당연히 서버(파이참)의 콘솔창에 출력되겠지.
이번에 반환한
return
이하의 값은 프론트 쪽에서 ‘콘솔창에 출력되는’ 방식으로 처리되게 되어있다.
실행 화면:
참고로
localhost:5000/test
페이지는 이렇게 생겼다:localhost:5000
페이지에서 버튼을 누르면서버 콘솔창(파이참의 콘솔)에는 이렇게:
웹 브라우저 콘솔창에는 이렇게:
뜨게 된다.
프론트 쪽과 서버 쪽 코드 정리
프론트 쪽과 서버 쪽 코드 정리
주석만 읽으시오.
프론트:
from flask import Flask, render_template, request, jsonify
app = Flask(__name__)
## 여러 return 형식:
# 1. 단순 문자열이 반환되고 브라우저 페이지에 그대로 출력되는 형식
@app.route('/mypage')
def mypage():
return 'This is Mypage~~!!!'
# 2. HTML코드가 문자열로 반환되고 브라우저 페이지에 잘 인코딩되어 출력되는 형식
@app.route('/mytest')
def home():
return '<button>버튼입니다. HTML 처리되어 진짜 버튼이 나오지요</button>'
# 3. templates라는 폴더에서 'index.html'파일을 찾아 브라우저 페이지에 출력하는 형식
@app.route('/')
def home():
return render_template('index.html')
# 4. '/test'라는 창구에 'GET' 요청이 오면: 이러저러한 처리를 수행하고, 응답(response)으로 이곳의 반환값이 주어지는 형식
@app.route('/test', methods=['GET'])
def test_get():
title_receive = request.args.get('title_give')
print(title_receive)
return jsonify({'result':'success', 'msg': '이 요청은 GET!'})
if __name__ == '__main__':
app.run('0.0.0.0',port=5000, debug=True)
서버:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<!--1. jQuery를 임포트해야 Ajax를 쓸 수 있다는 것 잊지 말기-->
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
<title>Document</title>
<script>
function hey() {
<!--2. 'url' 창구에 GET요청을 보낸다. response는 저(서버)쪽 해당 함수가 JSON 형태로 보낸 return값일텐데 그걸 브라우저 콘솔창에 출력한다.-->
$.ajax({
type: "GET",
url: "/test?title_give=봄날은간다",
data: {},
success: function (response) {
console.log(response)
}
})
}
</script>
</head>
<body>
<!--3. 버튼을 누르면 이 모든 일이 실행된다-->
<button onclick="hey()">나는 버튼!</button>
</body>
</html>
Flask - POST 요청과 응답
Flask - POST 요청과 응답
1. 프론트(index.html)에 Ajax로 POST 요청 심어주기
1. 프론트(index.html)에 Ajax로 POST 요청 심어주기
<script>
function hey() {
$.ajax({
type: "POST",
url: "/test",
data: {title_give: '봄날은간다'},
success: function (response) {
console.log(response)
}
})
}
</script>
2. 서버(app.py)에 POST 대응 API코드 만들기
2. 서버(app.py)에 POST 대응 API코드 만들기
# '/test' 창구에 POST 요청을 받았을 경우:
@app.route('/test', methods=['POST'])
def test_post():
title_receive = request.form['title_give']
print(title_receive)
return jsonify({'result':'success', 'msg': '요청을 잘 받았어요'})
- 왜 GET 요청 때는 data항목으로 키-값을 넘겨주지 않는 걸까? POST 때와는 무슨 차이지?
A. GET방식 때 URL 뒤에 데이터를 붙여 보내는 것과 달리 POST 방식 때는 따로 data항목에 담아서 보내는 이유는, 일단 데이터를 ‘보이지 않게’ 전달하게 된다는 점에 실마리가 있는 듯 하다.
Flask 기초 2탄 - 화성땅 공동구매
Flask 기초 2탄 - 화성땅 공동구매
필요 패키지: flask(서버 구축용), pymongo(DB 연결용), dnspython(?)
기본으로 제공된 템플릿에 적절한 GET과 POST 요청 주고받기 처리하기.
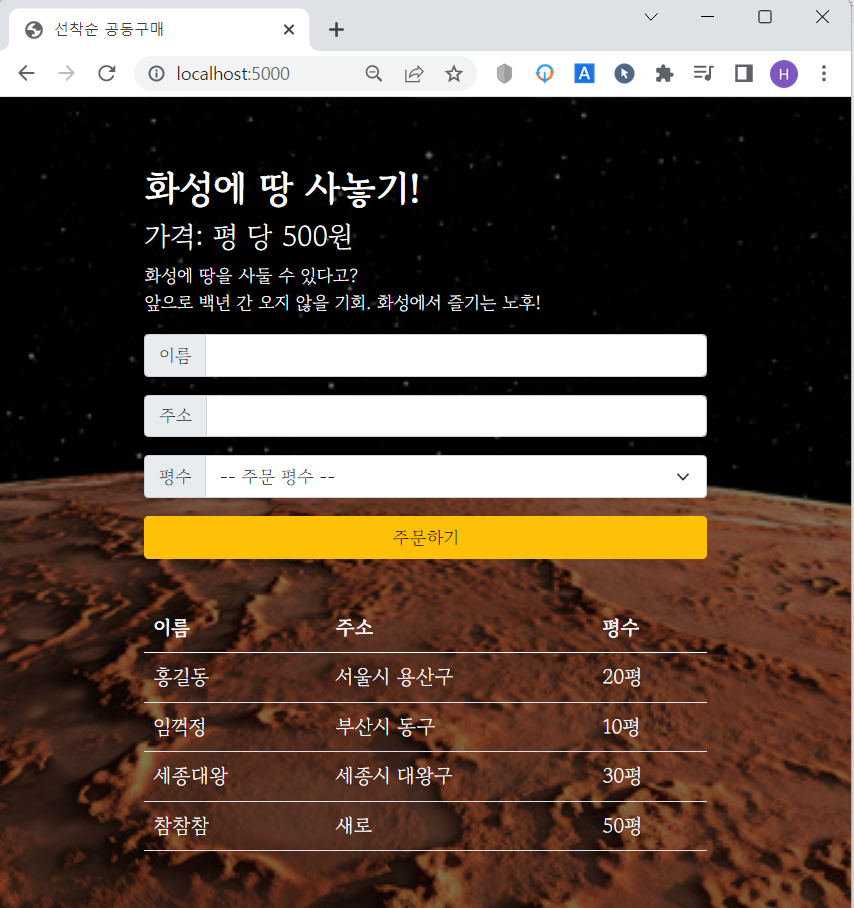
순서 요약 (이것만 보고 똑같이 짜볼 수 있기를):
- 필요 프로젝트 생성 (패키지 설치 포함)
- Flask 프로젝트용 기본 폴더 구조 만들기 - static 폴더, templates 폴더와 그 안의 index.html 파일, app.py 파일
- index.html와 app.py에 템플릿 복붙
- 양 쪽에 POST용, GET용 함수가 잘 짜여져 있는지(서버와 프론트가 잘 통신하는지) 간단히 확인
- 서버측(app.py) POST 내용 채워넣기 - 이 때 창구명과 약속 키(key)값 임의로 정함
- 프론트측(index.html) POST 내용 채워넣기 - ‘창구’에 약속된 ‘키’이름으로 값 전달. 이 때 마지막으로 페이지를 새로고침하도록 하는 코드를 넣는다
- 새 포스트를 하나 올려보고 DB에 잘 들어갔나 확인
- 서버측(app.py) GET 내용 채워넣기 - 그냥 DB에서 전부 불러와서, list()로 잘 감싸주고, JSON 형식으로 만들어서 반환해주면 된다
- 프론트측(index.html) GET 내용 채워넣기 - 받아온 응답을 순회하며 웹 페이지의 적절한 위치에 데이터 그려주기
- 웹 페이지를 새로고침해서 업데이트가 잘 되나 확인
POST (화성땅 주문 저장하기)
POST (화성땅 주문 저장하기)
1. 요청 정보 : URL=/mars
, 요청 방식 =POST
2. 클라(ajax) → 서버(flask) :name
,address
,size
3. 서버(flask) → 클라(ajax) : 메시지를 보냄 (주문 완료!)
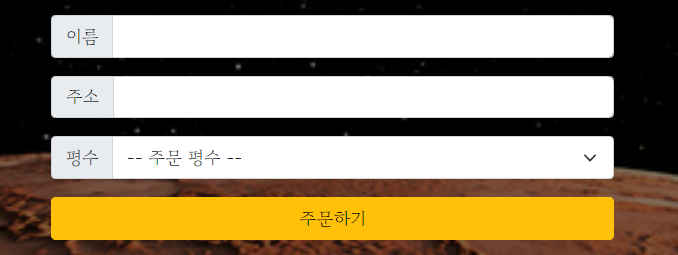
서버(app.py)측
서버(app.py)측
@app.route("/mars", methods=["POST"])
def web_mars_post():
name_received = request.form['name_give']
address_received = request.form['address_give']
size_received = request.form['size_give']
doc = {
'name': name_received,
'address': address_received,
'size': size_received,
}
db.mars.insert_one(doc)
return jsonify({'msg': '주문 완료!'})
프론트(index.html)측
프론트(index.html)측
function save_order() {
let name = $('#name').val();
let address = $('#address').val();
let size = $('#size').val(); // <select>태그도 .val()로 '선택된'옵션의 val을 추출할 수 있구나
$.ajax({
type: 'POST',
url: '/mars',
data: { name_give: name, address_give: address, size_give: size },
success: function (response) {
alert(response['msg'])
window.location.reload() // 페이지를 한 번 새로고침
}
});
}
이제 MongoDB Atlas에 가서 /dbsparta/mars 컬렉션을 확인해보면 데이터가 잘 들어와 있는 것을 확인할 수 있다.
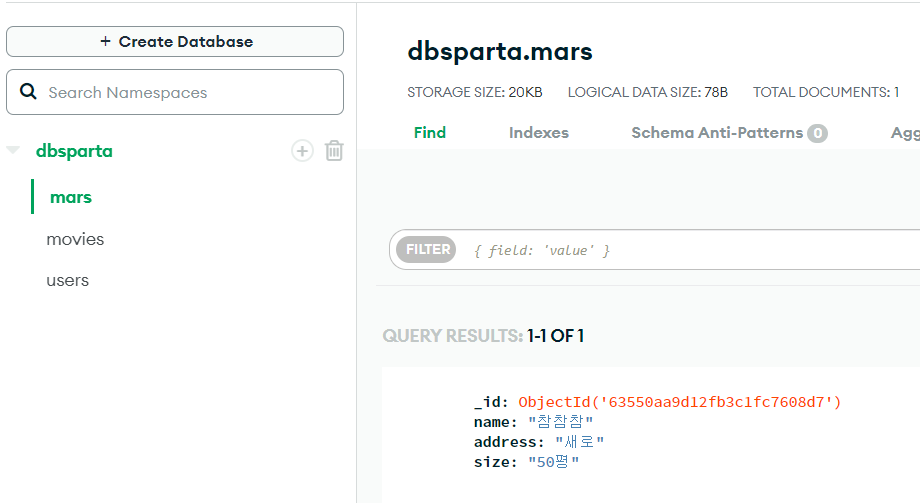
GET (화성땅 주문 보여주기)
GET (화성땅 주문 보여주기)
1. 요청 정보 : URL=/mars
, 요청 방식 =GET
2. 클라(ajax) → 서버(flask) : (없음) 3. 서버(flask) → 클라(ajax) : 전체 주문을 보내주기
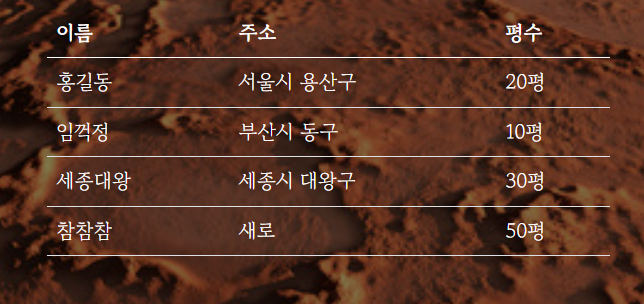
서버(app.py)측
서버(app.py)측
@app.route("/mars", methods=["GET"])
def web_mars_get():
# DB에서 데이터 가져오기
buyers_list = list(db.mars.find({}, {'_id':False}))
# 웹 브라우저에 보내주기
return jsonify({'msg': 'GET 연결 완료!', 'buyers_list': buyers_list})
프론트(index.html)측
프론트(index.html)측
function show_order() {
$.ajax({
type: 'GET',
url: '/mars',
data: {},
success: function (response) {
alert(response['msg'])
let buyers_list = response['buyers_list']
for (let i = 0; i < buyers_list.length; i++) {
let name = buyers_list[i]['name']
let address = buyers_list[i]['address']
let size = buyers_list[i]['size']
let temp_html = `<tr>
<td>${name}</td>
<td>${address}</td>
<td>${size}</td>
</tr>`
$('#buyers-list').append(temp_html)
}
}
});
}
이제 웹 페이지를 새로고침 했을 때 DB에 저장된 땅 구매자 데이터가 화면에 잘 나타나는 것을 확인할 수 있다.
언제 웹 페이지에서 ‘땅 구매자’ 목록이 업데이트 되게 될까?
언제 웹 페이지에서 ‘땅 구매자’ 목록이 업데이트 되게 될까?
// 프론트(index.html)측 크게 본 구조:
<script>
// #1. 페이지가 로딩되면 GET 요청을 한 번 불러옴
$(document).ready(function () {
show_order();
});
// #2. GET 요청: 서버에서 받은 응답(DB 데이터)을 이용해 웹페이지의 ‘땅 구매자 목록’ 항목을 업데이트함
function show_order() {
...
}
// #3. POST 요청: 서버에 신규 '땅 구매자' 데이터를 보내고, 페이지를 한 번 새로고침 함
function save_order() {
window.location.reload() // 페이지를 한 번 새로고침
}
</script>
페이지가 ‘로딩이 완료되는 때’의 두 가지 경우:
- 처음 페이지에 들어갔을 때: #1 → #2 (1번이 2번을 부름)
- 페이지를 새로고침했을 때: #3 → #1 → #2
따라서 위와 같이 코드를 짬으로써 ‘처음 페이지에 진입할 때와 포스팅이 될 때마다’ 땅 구매자 목록을 웹 페이지에 실시간으로 업데이트하는 싸이클이 완성되게 된다.
Flask 기초 3탄 - 영화 리뷰 카드
Flask 기초 3탄 - 영화 리뷰 카드
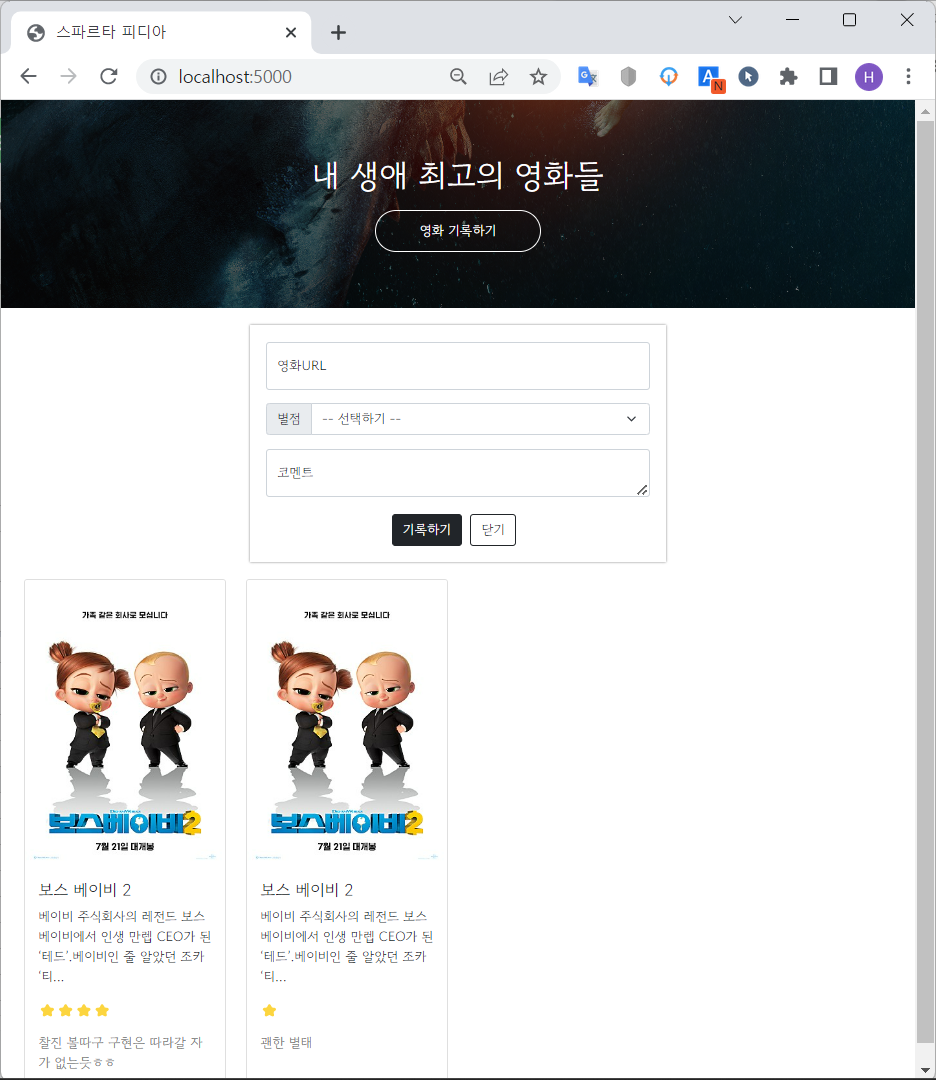
필요 패키지와 프로그래밍 순서는 위의 화성땅 공동구매와 같다.
다만 POST(기록하기)에 크롤링 기능이 추가되어야 하는 점과, 그에 따른 requests, bs4 패키지가 추가로 필요하다는 점만 다르다.
POST (영화 리뷰 카드 포스팅하기)
POST (영화 리뷰 카드 포스팅하기)
1. 요청 정보 : URL=/movie
, 요청 방식 =POST
2. 클라(ajax) → 서버(flask) :url
,star
,comment
3. 서버(flask) → 클라(ajax) : 메시지를 보냄 (포스팅 완료!)
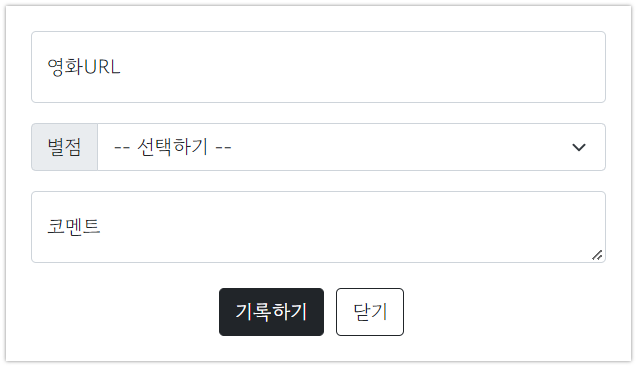
서버(app.py)측
서버(app.py)측
@app.route("/movie", methods=["POST"])
def movie_post():
url = request.form['url_give']
rate = request.form['rate_give']
comment = request.form['comment_give']
data = requests.get(url, headers=headers)
soup = BeautifulSoup(data.text, 'html.parser')
title = soup.select_one('meta[property="og:title"]')['content']
img = soup.select_one('meta[property="og:image"]')['content']
desc = soup.select_one('meta[property="og:description"]')['content']
doc = {
'url': url,
'image': img,
'title': title,
'description': desc,
'rate': rate,
'comment': comment,
}
db.movieCards.insert_one(doc)
return jsonify({'msg': '영화 카드 포스팅 완료!'})
<meta>태그 중에 property=”og:title”인 속성을 갖는 애(들)을 선택하기: meta[property="og:title"]
프론트(index.html)측
프론트(index.html)측
function posting() {
let url = $('#url').val()
let rate = $('#star').val()
let comment = $('#comment').val()
$.ajax({
type: 'POST',
url: '/movie',
data: { 'url_give': url, rate_give: rate, comment_give: comment },
success: function (response) {
alert(response['msg'])
window.location.reload()
}
});
}
GET (영화 리뷰 카드 보여주기)
GET (영화 리뷰 카드 보여주기)
1. 요청 정보 : URL=/movie
, 요청 방식 =GET
2. 클라(ajax) → 서버(flask) : (없음) 3. 서버(flask) → 클라(ajax) : 전체 영화를 보내주기
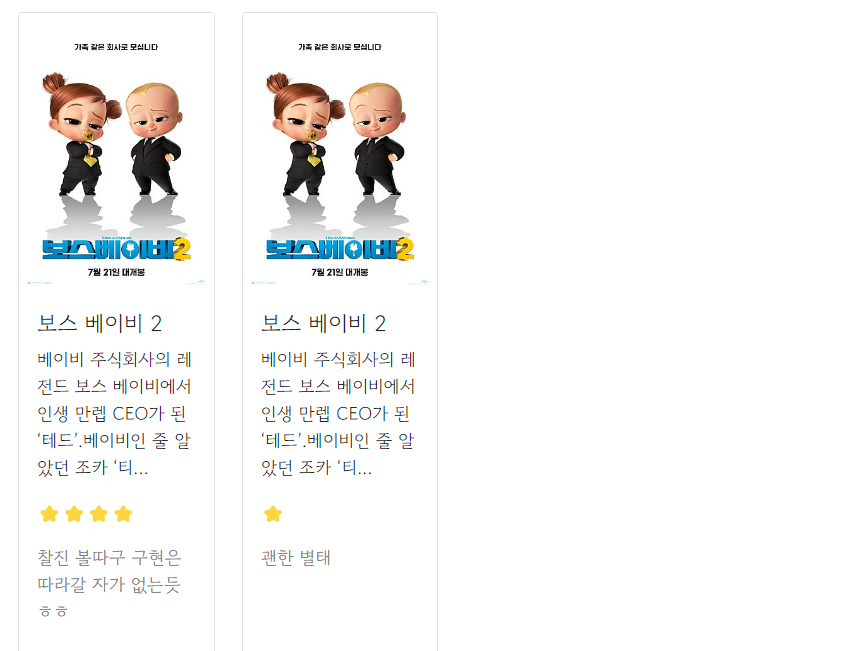
서버(app.py)측
서버(app.py)측
@app.route("/movie", methods=["GET"])
def movie_get():
movies_list = list(db.movieCards.find({}, {'_id':False}))
return jsonify({'msg': 'GET 연결 완료!', 'movies_list': movies_list})
프론트(index.html)측
프론트(index.html)측
function listing() {
$.ajax({
type: 'GET',
url: '/movie',
data: {},
success: function (response) {
alert(response['msg'])
$('#cards-box').empty()
let movies_list = response['movies_list']
for (let i = 0; i < movies_list.length; i++) {
let image = movies_list[i]['image']
let title = movies_list[i]['title']
let desc = movies_list[i]['description']
let rate = '⭐'.repeat(movies_list[i]['rate'])
let comment = movies_list[i]['comment']
let temp_html = `<div class="col">
<div class="card h-100">
<img src=${image}
class="card-img-top">
<div class="card-body">
<h5 class="card-title">${title}</h5>
<p class="card-text">${desc}</p>
<p>${rate}</p>
<p class="mycomment">${comment}</p>
</div>
</div>
</div>`
$('#cards-box').append(temp_html)
}
}
})
}
Flask 기초 4탄 - 숙제 팬명록
Flask 기초 4탄 - 숙제 팬명록
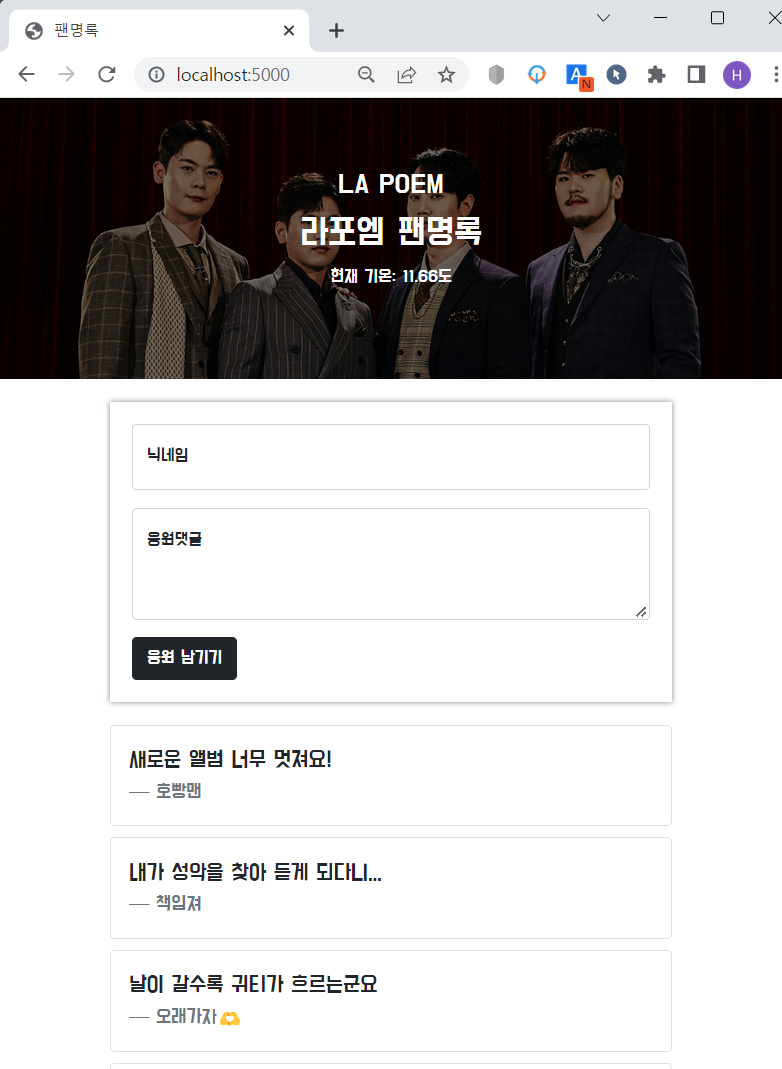
필요 패키지: flask(서버 구축용), pymongo(DB 연결용), dnspython(?)
1주차에 완성한 팬명록 페이지에 적절한 GET과 POST 요청 주고받기 처리하여 완성하기:
1) 응원 남기기(POST): 정보 입력 후 '응원 남기기' 버튼클릭 시 주문목록에 추가 2) 응원 보기(GET): 페이지 로딩 후 하단 응원 목록이 자동으로 보이기
POST (팬명록 저장하기)
POST (팬명록 저장하기)
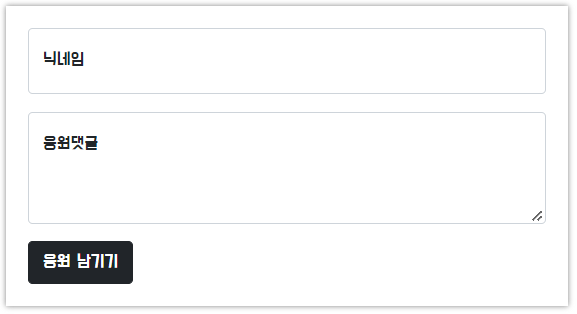
서버(app.py)측
서버(app.py)측
@app.route("/homework", methods=["POST"])
def homework_post():
name_received = request.form['name_give']
comment_received = request.form['comment_give']
doc = {
'name': name_received,
'comment': comment_received,
}
db.homework.insert_one(doc)
return jsonify({'msg': '코멘트 등록 완료'})
프론트(index.html)측
프론트(index.html)측
function save_comment() {
// '코멘트'나 '닉네임'칸 중 하나라도 비어있으면 입력해달라는 alert() 띄우기
let name = $('#name').val()
let comment = $('#comment').val()
if (!name) {
alert('닉네임을 작성해주세요!')
return
}
if (!comment) {
alert('코멘트 내용을 작성해주세요!')
return
}
// Ajax로 POST 요청 보내기
$.ajax({
type: 'POST',
url: '/homework',
data: { 'name_give': name, 'comment_give': comment },
success: function (response) {
alert(response['msg'])
window.location.reload()
}
})
}
GET (팬명록 보여주기)
GET (팬명록 보여주기)
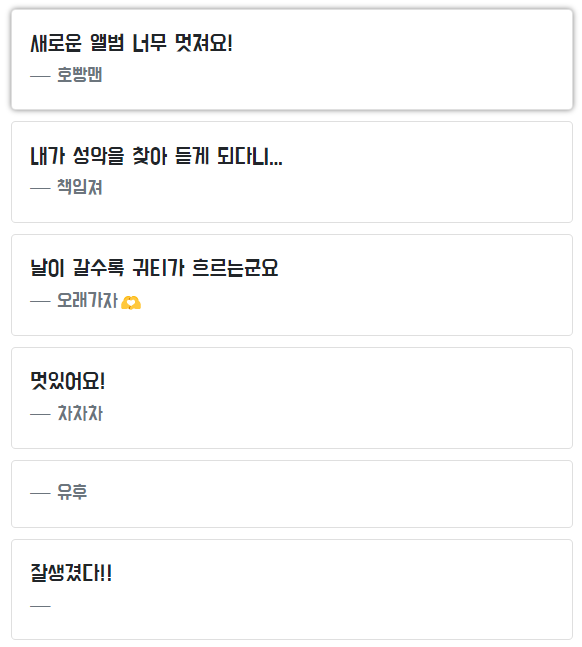
서버(app.py)측
서버(app.py)측
@app.route("/homework", methods=["GET"])
def homework_get():
comments_list = list(db.homework.find({}, {'_id':False}))
return jsonify({'msg': '코멘트 불러오기 완료!', 'comments_list': comments_list})
프론트(index.html)측
프론트(index.html)측
function show_comment() {
// $('#comment-cards').empty()
$.ajax({
type: 'GET',
url: '/homework',
data: {},
success: function (response) {
alert(response['msg'])
let comments_list = response['comments_list']
for (let i = 0; i < comments_list.length; i++) {
let name = comments_list[i]['name']
let comment = comments_list[i]['comment']
let temp_html = `<div onclick="delete_comment()" class="card">
<div class="card-body">
<blockquote class="blockquote mb-0">
<p>${comment}</p>
<footer class="blockquote-footer">${name}</footer>
</blockquote>
</div>
</div>`
$('#comment-cards').append(temp_html)
}
}
})
}
DELETE 기능도 구현해보려 했지만 실패했다. ‘클릭한 이 카드’의 코멘트와 닉네임을 얻어올 수가 없어서. 실패하고 주석처리 해놓은 부분까지 합친 전체 코드는 아래 토글을 확인하자.
내 팬명록 전체 코드
내 팬명록 전체 코드
서버측(app.py)
서버측(app.py)
## 서버로의 연결 세팅
from flask import Flask, render_template, request, jsonify
app = Flask(__name__)
## 클라우드 DB로의 연결 세팅
from pymongo import MongoClient
client = MongoClient('mongodb+srv://<ID>:<PASSWORD>@cluster0.ecmqblf.mongodb.net/?retryWrites=true&w=majority')
db = client.dbsparta
@app.route('/')
def home():
return render_template("04index_my.html")
@app.route("/homework", methods=["POST"])
def homework_post():
name_received = request.form['name_give']
comment_received = request.form['comment_give']
doc = {
'name': name_received,
'comment': comment_received,
}
db.homework.insert_one(doc)
return jsonify({'msg': '코멘트 등록 완료'})
@app.route("/homework", methods=["GET"])
def homework_get():
comments_list = list(db.homework.find({}, {'_id':False}))
return jsonify({'msg': '코멘트 불러오기 완료!', 'comments_list': comments_list})
# @app.route("/homework2", methods=["POST"])
# def homework_delete():
# target_name = request.form['name']
# target_comment = request.form['comment']
# print(target_name, target_comment)
# print("뭐라도 출력해봐...")
# print(request.form)
# db.homework.delete_one({'name': target_name, 'comment': target_comment})
# return jsonify({'msg': '해당 코멘트를 삭제하였습니다'})
if __name__ == '__main__':
app.run('0.0.0.0', port=5000, debug=True)
프론트측(index.html)
프론트측(index.html)
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/css/bootstrap.min.css" rel="stylesheet"
integrity="sha384-EVSTQN3/azprG1Anm3QDgpJLIm9Nao0Yz1ztcQTwFspd3yD65VohhpuuCOmLASjC" crossorigin="anonymous">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
<script src="https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/js/bootstrap.bundle.min.js"
integrity="sha384-MrcW6ZMFYlzcLA8Nl+NtUVF0sA7MsXsP1UyJoMp4YLEuNSfAP+JcXn/tWtIaxVXM"
crossorigin="anonymous"></script>
<title>팬명록</title>
<link href="https://fonts.googleapis.com/css2?family=Do+Hyeon&display=swap" rel="stylesheet">
<style>
* {
font-family: 'Do Hyeon', sans-serif;
}
.mytitle {
width: 100%;
height: 250px;
/* 배경화면 이미지 삼형제 */
background-image: linear-gradient(0deg, rgba(0, 0, 0, 0.5), rgba(0, 0, 0, 0.5)), url(https://image.genie.co.kr/Y/IMAGE/IMG_MUZICAT/IV2/Genie_Magazine/10378/Mgz_Sub_EVT_20210623163827.jpg);
background-size: cover;
background-position: center 40%;
color: white;
/* 상자(나) 안 내용물 가운데 정렬하기 4형제 */
display: flex;
flex-direction: column;
justify-content: center;
align-items: center;
}
.mypost {
box-shadow: 0px 0px 5px 0px gray;
max-width: 500px;
width: 95%;
margin: 20px auto 20px auto;
padding: 20px;
}
.post-buttons {
margin-top: 15px;
}
.comment-cards {
max-width: 500px;
width: 95%;
margin: 10px auto;
}
.card {
margin: 10px auto 10px auto;
}
.card:hover {
box-shadow: 0px 0px 5px 0px gray;
}
</style>
<script>
// 페이지 로딩이 완료되면
$(document).ready(function() {
set_temp()
show_comment()
});
// Ajax가 GET방식으로 서울시 현재 기온을 읽어오고 표시한다.
function set_temp() {
$.ajax({
type: "GET",
url: "http://spartacodingclub.shop/sparta_api/weather/seoul",
data: {},
success: function (response) {
$('#temperature').text(response['temp']);
}
})
}
// POST
function save_comment() {
// '코멘트'나 '닉네임'칸 중 하나라도 비어있으면 입력해달라는 alert() 띄우기
let name = $('#name').val()
let comment = $('#comment').val()
if (!name) {
alert('닉네임을 작성해주세요!')
return
}
if (!comment) {
alert('코멘트 내용을 작성해주세요!')
return
}
// Ajax로 POST 요청 보내기
$.ajax({
type: 'POST',
url: '/homework',
data: { 'name_give': name, 'comment_give': comment },
success: function (response) {
alert(response['msg'])
window.location.reload()
}
})
}
// GET
function show_comment() {
// $('#comment-cards').empty()
$.ajax({
type: 'GET',
url: '/homework',
data: {},
success: function (response) {
alert(response['msg'])
let comments_list = response['comments_list']
for (let i = 0; i < comments_list.length; i++) {
let name = comments_list[i]['name']
let comment = comments_list[i]['comment']
let temp_html = `<div onclick="delete_comment()" class="card">
<div class="card-body">
<blockquote class="blockquote mb-0">
<p>${comment}</p>
<footer class="blockquote-footer">${name}</footer>
</blockquote>
</div>
</div>`
$('#comment-cards').append(temp_html)
}
}
})
}
// // $('.card').on('click', )
// // DELETE : 아무 카드를 누르면 '삭제하시겠습니까?' 띄우고 '확인'버튼과 '취소'버튼 중 선택
// function delete_comment() {
// let name = $(this).text() // children("p").text()
// let comment = $(this).children().text()
// if (confirm("해당 코멘트를 삭제하시겠습니까?")) {
// // '확인'버튼을 눌렀을 시:
// // 카드 영역마다 '누르기' 기능 심기
// $.ajax({
// type: 'POST',
// url: '/homework2',
// data: { name: name, comment: comment },
// success: function (response) {
// alert(response['msg'])
// window.location.reload()
// }
// })
// }
// }
</script>
</head>
<body>
<div class="mytitle">
<h3>LA POEM</h3>
<h1>라포엠 팬명록</h1>
<p>현재 기온: <span id="temperature">00.0</span>도</p>
</div>
<div class="mypost">
<div class="form-floating mb-3">
<input type="email" class="form-control" id="name" placeholder="name@example.com">
<label for="floatingInput">닉네임</label>
</div>
<div class="form-floating">
<textarea class="form-control" placeholder="Leave a comment here" id="comment"
style="height: 100px"></textarea>
<label for="floatingTextarea2">응원댓글</label>
</div>
<div class="post-buttons">
<button onclick="save_comment()" type="button" class="btn btn-dark">응원 남기기</button>
</div>
</div>
<div class="comment-cards" id="comment-cards">
<div class="card">
<div class="card-body">
<blockquote class="blockquote mb-0">
<p>새로운 앨범 너무 멋져요!</p>
<footer class="blockquote-footer">호빵맨</footer>
</blockquote>
</div>
</div>
<div class="card">
<div class="card-body">
<blockquote class="blockquote mb-0">
<p>내가 성악을 찾아 듣게 되다니...</p>
<footer class="blockquote-footer">책임져</footer>
</blockquote>
</div>
</div>
<div class="card">
<div class="card-body">
<blockquote class="blockquote mb-0">
<p>날이 갈수록 귀티가 흐르는군요</p>
<footer class="blockquote-footer">오래가자🫶</footer>
</blockquote>
</div>
</div>
</div>
</body>
</html>
정답 코드는 내 것과 다를 것이 없고 내가 덧붙인 기능들만 더 있을 뿐이므로 첨부를 생략했다.
참고:
스파르타 코딩클럽 > 웹개발 종합반(5주)의 영상 강의와 강의노트를 주로 참고함
가끔 구글링
Uploaded by N2T